Ruby Rails
Rails Presenter
Presenter Classes



It's time to beautify your Rails views

MetaPresenter is a 100% free Ruby gem for view presenter classes.
MetaPresenter is a 100% free Ruby gem for view presenter classes. Pull yourself out of the vortex of scattered logic.
To install just add to your Gemfile and bundle:
gem 'meta_presenter'
$ bundle install

Learn More


MetaPresenter

How does it work?

Each action gets its own Presenter class that the view calls with presenter.method_name . Presenters can also delegate to objects or controller methods.


Decompose your view logic into tight, easily-testable classes
In 4 simple steps
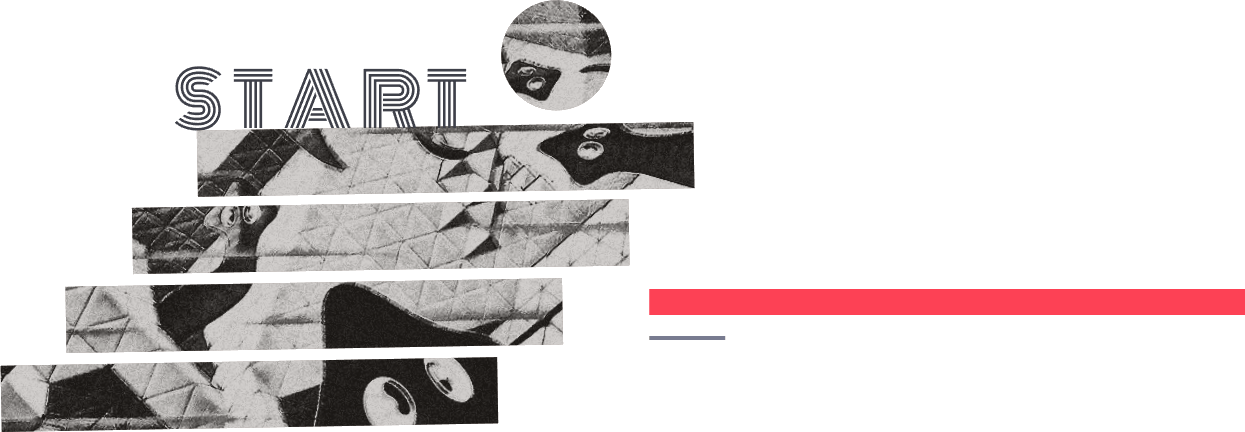
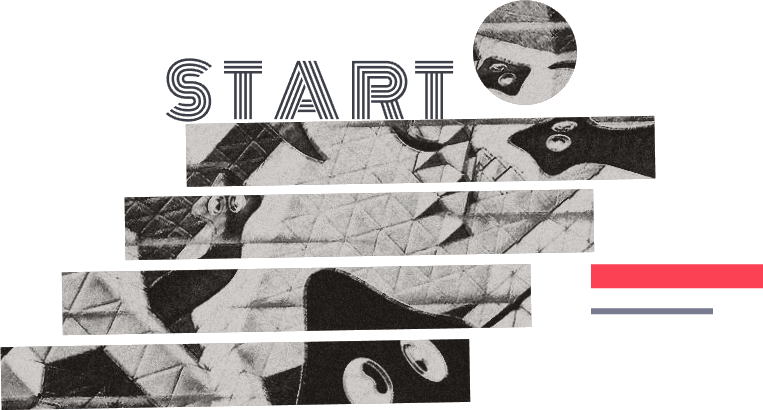

Include in your controller
Including MetaPresenter::Helpers
gives you access to the presenter
method within your views.
# app/presenters/application_presenter.rb
class ApplicationController < ActionController::Base
include MetaPresenter::Helpers
end
# Works with mailers, too!
class ApplicationMailer < ActionMailer::Base
include MetaPresenter::Helpers
end

Create a presenter class
Your presenter class is scoped to an individual view. No more chaos of global helper methods!
# app/presenters/pages/home_presenter.rb
class Pages::HomePresenter < PagesPresenter
# presenter.email, presenter.last_login_at or any
# other method not already defined will delegate
# to the current_user
delegate_all_to :current_user
# presenter.greeting in views
def greeting
"Hello, #{current_user.name}"
end
end

Write clean views with the presenter object
Now you can use presenter.whatever
in your views and it will delegate to the presenter.
<h1>Home</h1>
<p><%= presenter.greeting %></p>
<p>Last login <%= presenter.last_login_at %></p>

Test your presenter class
You can test presenters just like any other class. Don't be afraid to test every permutation because unlike system specs they run blazing fast!
describe Pages::HomePresenter do
let(:user) { User.new(name: "Mario") }
let(:controller) do
OpenStruct.new(current_user: user)
end
let(:presenter) do
described_class.new(controller)
end
describe '#hello' do
subject { presenter.hello }
it { is_expected.to eql("Hello, Mario") }
end
end
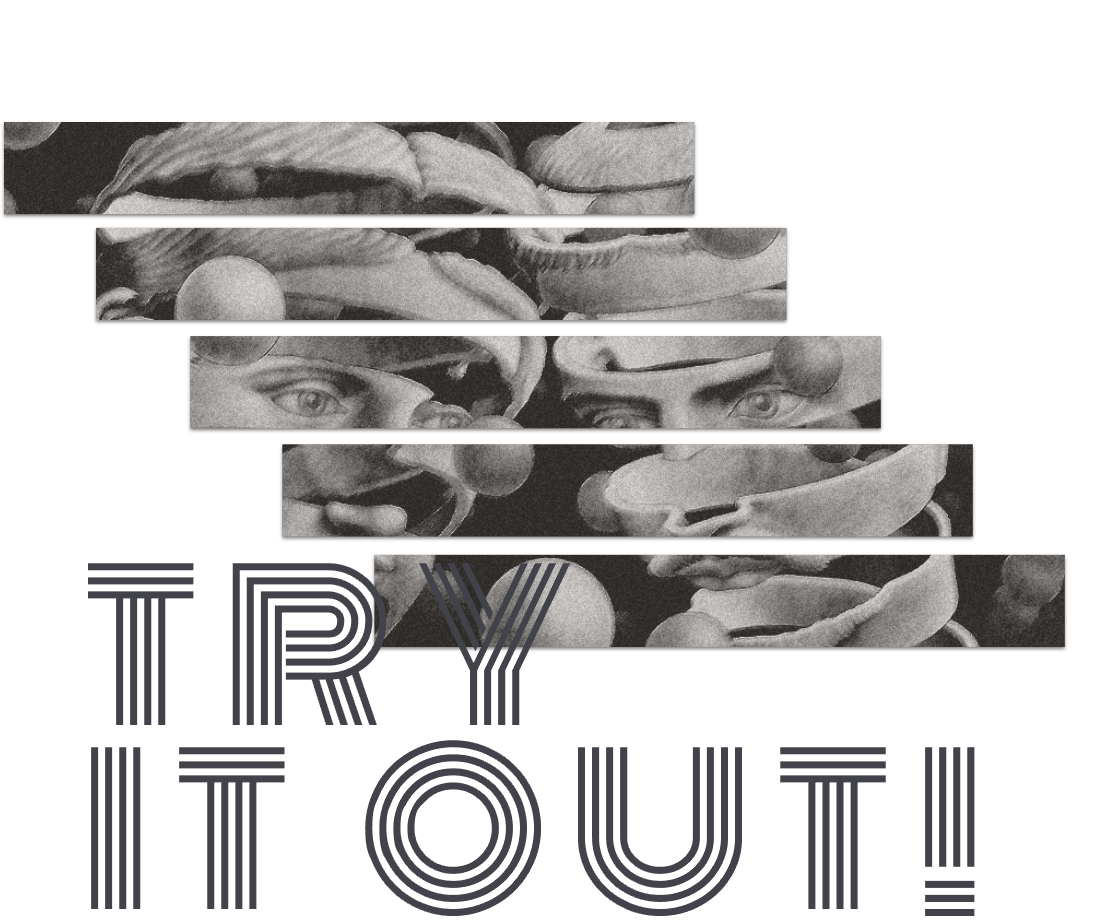



Are you ready to
Give it a try?


If you're ready to start beautifying your views, visit the GitHub page for setup instructions.